Signing Git commits with GPG
This recipe will show you how to digitally sign Git commits and RPM packages using a GNU Privacy Guard (GPG) keypair. A GPG keypair consists of two parts: the public key and the private key.
This is done by creating a GPG keypair and using it to sign Git commits and RPM packages.
Getting ready
In order to work with GPG and Git, you first need to install a few packages. Normally GPG is installed by default when the package gnupg2 is installed.
Git should be installed using dnf install git -y.
How to do it…
The first step is to create a GPG key if you do not already have one. This key will be used to sign both your Git commits and RPM packages. To work with the GPG key, you can use the gpg or gpg2 commands; both are the same thing, as gpg links to gpg2.
The GPG key is created via the command line:
[erik@ol8 ~]$ gpg2 –gen-key
The command will ask for some information, mainly your real name and email address. After you enter the information and continue, it will ask you to set a passphrase to use the key. Do not forget the passphrase! If you do, the key will become unusable and you will lose all data encrypted with the key. You can choose not to use a passphrase, but if you do this, you will be asked to verify this several times. The output of the key creation should be similar to the following screenshot:
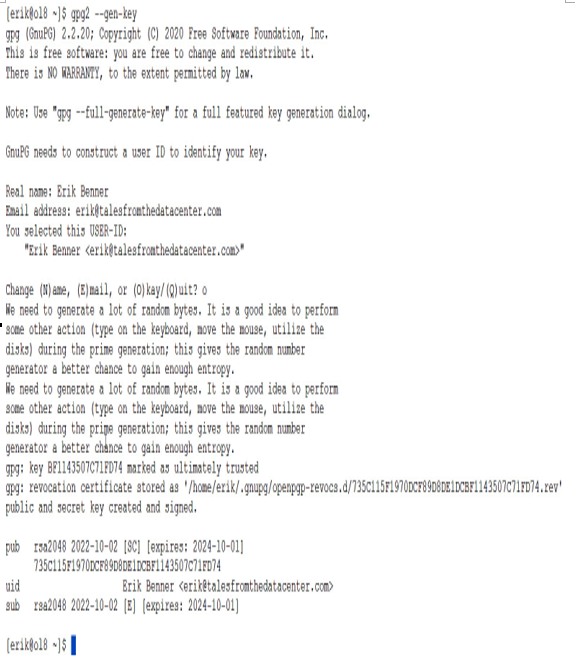
Figure 9.1 – GPG keys created
Optionally, you can use the –full-generate-key option, which can be used to create keys stored on hardware devices, among other uses.
Once the key is created, it is put in your keyring, the pubring.kbx file in the .gnupg directory in your home directory. To see all the keys in the file, run the gpg2 –list-keys –keyid-format=long command.
The gpg –list-secret-keys command is used to list the secret keys (i.e., private keys) stored in your GPG keyring. Running the command will show output similar to the following figure for GPG keys:
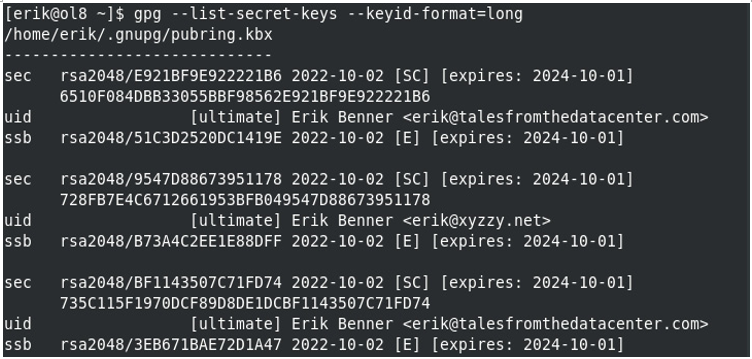
Figure 9.2 – GPG keys
The output gives several columns of information for each key:
• sec: This column indicates that the key is a secret key.
• rsa2048/4096/…: This column shows the algorithm and key length used by the key. For example, rsa2048 indicates that the key uses the RSA algorithm with a key length of 2,048 bits. If the long format is used, this will also contain the key fingerprint after the key length.
• [creation date]: This column shows the date on which the key was created.
• [expiration date]: If the key has an expiration date set, it will be shown in this column. If not, this column will be empty.
• [uid]: This column shows the user ID associated with the key. This is usually the name and email address of the key’s owner.
• [ssb]: This column indicates that the key has a corresponding subkey (i.e., a separate key used for encryption, signing, or authentication).
• [expires]: If the subkey has an expiration date set, it will be shown in this column. If not, this column will be empty.
The key fingerprint is a shortened digital representation of the key. It allows other people to validate that your public key was sent with no tampering. The long key ID format for the GPG command is a 16-character hexadecimal string that uniquely identifies a public or private key in GPG. It is often used to refer to a specific key when working with GPG.
The long key ID is derived from the full 40-character fingerprint of the key. The fingerprint is a cryptographic hash of the key’s public key material, and it is used to verify the authenticity of the key. The long key ID is the last 16 characters of the fingerprint.
Now that you have a key, we need to import it into Git. In the following example, we will use the key E921BF9E922221B6. To import the key, we will use the following command:
git config –global user.signingkey E921BF9E922221B6
Then, we can set Git to sign all commits by default using the following command:
git config –global commit.gpgsign true
How it works…
Git can sign both commits and tags using the GPG key. Be careful with Git though, as it isn’t consistent. git tag uses -s to sign tags, but git commit uses -s to add the developers certificate of origin (DCO) signed-off line to the commit text and -S actually signs the commit using GPG.
To set up a new tag, we will use the Git tag -s $TAG -m $TAG_DESCRIPTION:
$ git tag -s v1.0 -m ‘Version 1.0 tag’
You need a passphrase to unlock the secret key for
user: “Erik Benner [email protected]“
2048-bit RSA key, ID 922221B6, created 2024-04-01
NOTE
You can also set tag.gpgsign either globally or per-repo to ensure all tags are automatically signed.
Next, we can show the tag with git show v1.0:
$ git show v1.0
tag v1.0
Tagger: Erik Benner
Date: Sun April 2 215:24:41 2023 -2300
Version 1.0 tag
—–BEGIN PGP SIGNATURE—–
Version: GnuPG v1
JHGSksdhj847yskKJHSnd874tlKJAHGS32674GJHSAD784ghsjkd7*&^ADDXGFdgj3kjj hde018GDKjskhdh8737ybdhajkjkjhsadfs7892987812mmxnbcbsd8JASJ74845jfhHA SHDepkjahZXkjs83828732ZMKDjh92yaskjagZKDSJHGHHD&=7213kj4ha,,jhsad10al qpdif9839928hdkask
=EFTF
—–END PGP SIGNATURE—–
commit 7fhs49185hflvmsgd742825hhabzb182ufnc8
Author: Erik Benner [email protected]
Date: Sun Apr 2 12:52:11 2023 -2300
Change version number
After this, when we check code using Git, add a -S option to the command to automatically sign the commit with the tag:
git comit -a -S -m “comment goes here”
NOTE
This is not needed if commit.gpgsign=true is set either globally or in the active Git repo.